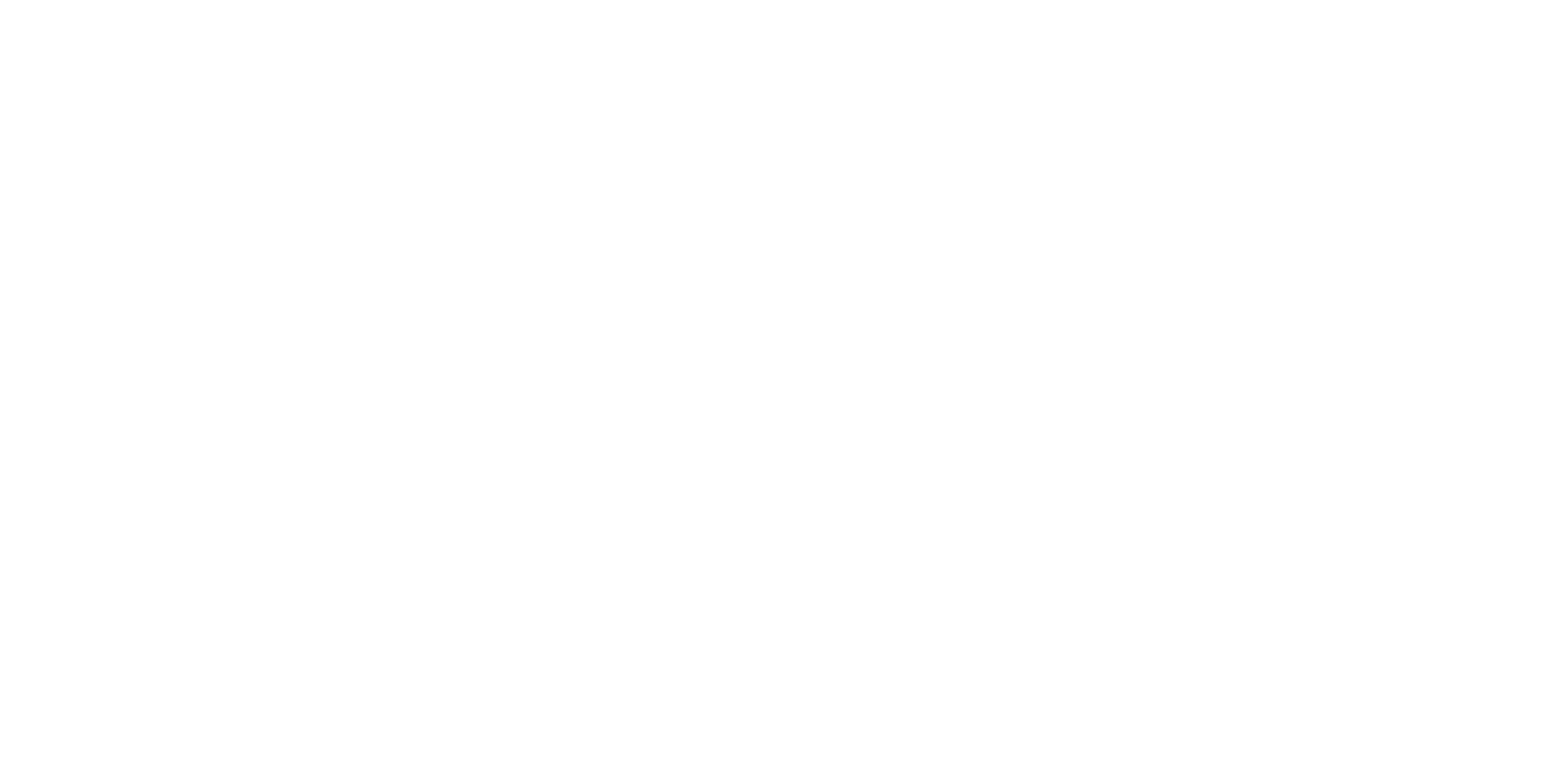
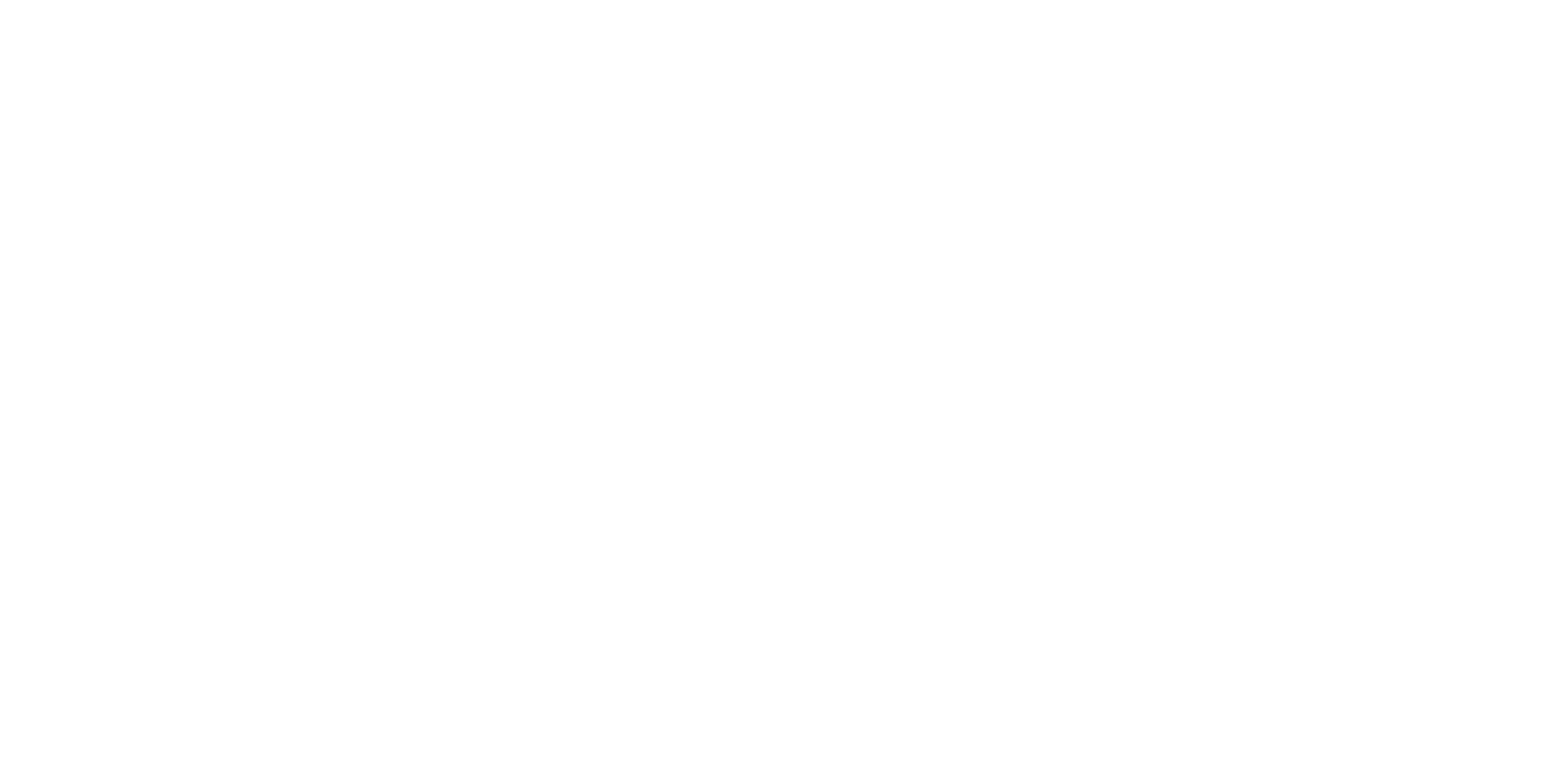
Guides
Error Handling
In this guide, you'll learn how to implement robust error handling strategies in your Rapture application to provide a smooth and user-friendly experience.
Introduction to Error Handling
Errors are an inevitable part of software development. Whether they're caused by invalid user input, server issues, or unexpected scenarios, handling errors properly is essential to ensure that your application remains reliable and user-friendly.
To implement basic error handling in your Rapture app, follow these steps:
Identify Potential Errors: Identify the areas in your code where errors can occur. This could be during data validation, API calls, or complex computations.
Use Try-Catch Blocks: Wrap the code that could potentially throw an error within a
try
block. Use thecatch
block to handle the error gracefully.
javascript
// api.js
export async function fetchMessages(conversationId, page = 1, limit = 10) {
const response = await fetch(
`/api/messages?conversation_id=${conversationId}&page=${page}&limit=${limit}`
);
if (!response.ok) {
throw new Error('Failed to fetch messages');
}
const data = await response.json();
return data;
}
Copy
Logging errors is crucial for diagnosing issues and improving your app's stability. Here's how you can log errors effectively:
Use a Logging Library: Utilize a logging library to record errors in your backend or frontend. Common libraries include Winston (Node.js) and console.error (browser).
Include Contextual Information: When logging an error, include contextual information such as the user's ID, the endpoint causing the error, or any relevant data.
javascript
// api.js
export async function fetchMessages(conversationId, page = 1, limit = 10) {
const response = await fetch(
`/api/messages?conversation_id=${conversationId}&page=${page}&limit=${limit}`
);
if (!response.ok) {
throw new Error('Failed to fetch messages');
}
const data = await response.json();
return data;
}
Copy
When an error occurs, providing users with helpful information can prevent frustration. Here's how you can display user-friendly errors:
Create an Error Component: Design a reusable error component that can display error messages to users.
Catch and Display Errors: In your application's UI, catch errors at a high level and render the error component with the appropriate error message.
javascript
// api.js
export async function fetchMessages(conversationId, page = 1, limit = 10) {
const response = await fetch(
`/api/messages?conversation_id=${conversationId}&page=${page}&limit=${limit}`
);
if (!response.ok) {
throw new Error('Failed to fetch messages');
}
const data = await response.json();
return data;
}
Copy
For more advanced error handling techniques, consider:
Custom Error Classes: Create custom error classes to differentiate and handle different types of errors.
Global Error Handlers: Implement global error handlers to catch unhandled errors and prevent app crashes.
Retry Mechanisms: Implement retry logic for transient errors, such as network timeouts.
Log errors with contextual information to aid debugging.
Provide clear error messages that guide users on resolving the issue.
Implement a graceful error-handling strategy to prevent app crashes.